Description
Allows creating CommandLine applications using Microsoft.Extensions.CommandLineUtils together with DI, Configuration and Logging in a convenient way similar to AspNetCore Hosting.
DarkXaHTeP.CommandLine alternatives and similar packages
Based on the "CLI" category.
Alternatively, view DarkXaHTeP.CommandLine alternatives based on common mentions on social networks and blogs.
-
Command Line Parser
The best C# command line parser that brings standardized *nix getopt style, for .NET. Includes F# support -
Fluent Command Line Parser
A simple, strongly typed .NET C# command line parser library using a fluent easy to use interface -
DotMake Command-Line
Declarative syntax for System.CommandLine via attributes for easy, fast, strongly-typed (no reflection) usage. Includes a source generator which automagically converts your classes to CLI commands and properties to CLI options or CLI arguments. -
NFlags
Simple yet powerfull library to made parsing CLI arguments easy. Library also allow to print usage help "out of box". -
Tamar.ANSITerm
“ANSITerm” provides ANSI escape codes and true color formatting for .NET Core's Console on Linux terminals.
InfluxDB - Power Real-Time Data Analytics at Scale
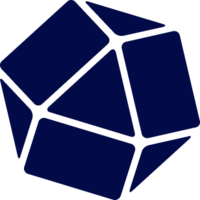
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of DarkXaHTeP.CommandLine or a related project?
README
DarkXaHTeP.CommandLine
Allows creating CommandLine applications using Microsoft.Extensions.CommandLineUtils together with DI, Configuration and Logging in a convenient way similar to AspNetCore Hosting.
NuGet
Build
Getting Started
To use the library you need to go through 2 simple steps:
Define Startup class similar to Asp.Net Core:
public class Startup
{
public void Configure(IApplicationBuilder app)
{
app.OnExecute(async () =>
{
Console.WriteLine("Hello World")
return 0;
});
}
}
Create CommandLine Host using CommandLineHostBuilder and run it:
static class Program
{
static int Main(string[] args)
{
ICommandLineHost host = new CommandLineHostBuilder()
.UseStartup<Startup>()
.Build();
return host.Run(args);
}
}
The application will print "Hello World" to console output and exit with 0 code.
Release notes
For release notes please see CHANGELOG.md
Startup class
Same as Asp.Net Core Startup, CommandLine Startup can have two methods:
void Configure(IApplicationBuilder app)
is required. This is where application
arguments and commands configuration happens using IApplicationBuilder.
void ConfigureServices(IServiceCollection services)
is optional. Allows configuring Dependency Injection
by providing access to application's IServiceCollection. This method is invoked prior to Configure
IApplicationBuilder interface
This interface provides a set of methods for configuring application. These methods are similar to provided by Microsoft.Extensions.CommandLineUtils (actually this library uses CommandLineUtils internally, however this may change in future due to the fact that CommandLineUtils is no longer supported).
IServiceProvider ApplicationServices { get; }
gives access to services registered in application
IApplicationBuilder Parent { get; }
contains link to parent IApplicationBuilder. Is null for the root builder
CommandArgument Argument(string name, string description, bool multipleValues = false);
allows to define command argument
IApplicationBuilder Command (string name, Action<IApplicationBuilder> configure, bool throwOnUnexpectedArg = true);
allows to define command
void OnExecute (Func<System.Threading.Tasks.Task<int>> invoke);
void OnExecute (Func<int> invoke);
allows to define root or command execution callback
CommandOption Option (string template, string description, CommandOptionType optionType);
allows to define option
void ShowHelp (string commandName = null);
shows help for the particular command or a whole app. Could be used in execute callbacks
Example:
var greeter = app.ApplicationServices.GetRequiredService<IGreeter>();
app.OnExecute(() => {
app.ShowHelp();
return 0;
});
app.Command("greet", command => {
var casualOption = command.Option("-c|--casual", "This greeting is informal/casual", CommandOptionType.NoValue);
var nameArgument = command.Argument("<name1 name2>", "Names of persons to greet separated with space", true);
command.OnExecute(async () => {
string names = nameArgument.Values;
bool isCasual = casualOption.HasValue();
string greeting = await greeter.GetGreetingAsync(isCasual);
greeter.GreetAll(greeting, names);
return 0;
});
});
CommandLineHostBuilder class
This class is similar to WebHostBuilder
from Asp.Net Core and allows to setup application configuration,
logging and arguments parsing strategy before execution.
ICommandLineHostBuilder ConfigureAppConfiguration(Action<IConfigurationBuilder> configureDelegate);
ICommandLineHostBuilder ConfigureAppConfiguration(Action<CommandLineHostBuilderContext, IConfigurationBuilder> configureDelegate);
allows to configure application configuration
ICommandLineHostBuilder ConfigureLogging(Action<ILoggingBuilder> configureLogging);
ICommandLineHostBuilder ConfigureLogging(Action<CommandLineHostBuilderContext, ILoggingBuilder> configureLogging);
allows to configure logging
ICommandLineHostBuilder UseContentRoot(string contentRoot);
allows to redefine content root that will be used for resolving relative paths (e.g. path to json configuration file if used)
ICommandLineHostBuilder UseStartup<TStartup>() where TStartup : class;
sets Startup class to use. This method is required to call because no automatic Startup discovery is implemented
ICommandLineHostBuilder AllowUnexpectedArgs();
changes argument parsing to be less strict and not throw on unexpected arguments
ICommandLineHost Build();
builds command line host
ICommandLineHostBuilder ConfigureServices(Action<IServiceCollection> configureServices);
ICommandLineHostBuilder ConfigureServices(Action<CommandLineHostBuilderContext, IServiceCollection> configureServices);
allows to define additional services. Could be used in extension methods to extend behaviour
Example:
static class Program
{
static int Main(string[] args)
{
ICommandLineHost host = new CommandLineHostBuilder()
.UseContentRoot(Directory.GetCurrentDirectory())
.ConfigureLogging(logging =>
{
logging
.AddConsole()
.AddDebug();
})
.ConfigureAppConfiguration(config =>
{
config
.AddJsonFile("appsettings.json")
.AddConsul("application")
.AddEnvironmentVariables();
})
.UseStartup<Startup>()
.AllowUnexpectedArgs()
.Build();
return host.Run(args);
}
}
Dependency Injection
It is possible to inject dependencies either through constructor of Startup
class or Configure
method,
however only built-in dependencies (IConfiguration
, ICommandLineEnvironment
, ILogger<>
etc.) are available through constructor
because ConfigureServices
method is not yet called.
Example:
public class Startup
{
public Startup(
IConfiguration config,
ICommandLineEnvironment environment,
ILogger<Startup> logger
)
{}
public void Configure(IApplicationBuilder app, IMyService service)
{
}
public void ConfigureServices(IServiceCollection services)
{
services.AddSingleton<IMyService, MyService>();
}
}
Acknowledgements
This library is highly inspired by AspNet.Core Hosting from Microsoft
Contribution
Feel free to ask questions and post bugs/ideas in the issues, as well as send pull requests.
*Note that all licence references and agreements mentioned in the DarkXaHTeP.CommandLine README section above
are relevant to that project's source code only.