Enums.NET alternatives and similar packages
Based on the "Misc" category.
Alternatively, view Enums.NET alternatives based on common mentions on social networks and blogs.
-
Polly
Polly is a .NET resilience and transient-fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner. From version 6.0.1, Polly targets .NET Standard 1.1 and 2.0+. -
Humanizer
Humanizer meets all your .NET needs for manipulating and displaying strings, enums, dates, times, timespans, numbers and quantities -
Coravel
Near-zero config .NET library that makes advanced application features like Task Scheduling, Caching, Queuing, Event Broadcasting, and more a breeze! -
Hashids.net
A small .NET package to generate YouTube-like hashes from one or many numbers. Use hashids when you do not want to expose your database ids to the user. -
Scientist.NET
A .NET library for carefully refactoring critical paths. It's a port of GitHub's Ruby Scientist library -
WorkflowEngine
WorkflowEngine.NET - component that adds workflow in your application. It can be fully integrated into your application, or be in the form of a specific service (such as a web service). -
HidLibrary
This library enables you to enumerate and communicate with Hid compatible USB devices in .NET. -
DeviceId
A simple library providing functionality to generate a 'device ID' that can be used to uniquely identify a computer. -
Warden
Define "health checks" for your applications, resources and infrastructure. Keep your Warden on the watch. -
Aeron.NET
Efficient reliable UDP unicast, UDP multicast, and IPC message transport - .NET port of Aeron -
ByteSize
ByteSize is a utility class that makes byte size representation in code easier by removing ambiguity of the value being represented. ByteSize is to bytes what System.TimeSpan is to time. -
Mediator.Net
A simple mediator for .Net for sending command, publishing event and request response with pipelines supported -
DeviceDetector.NET
The Universal Device Detection library will parse any User Agent and detect the browser, operating system, device used (desktop, tablet, mobile, tv, cars, console, etc.), brand and model. -
https://github.com/minhhungit/ConsoleTableExt
A fluent library to print out a nicely formatted table in a console application C# -
Valit
Valit is dead simple validation for .NET Core. No more if-statements all around your code. Write nice and clean fluent validators instead! -
FormHelper
ASP.NET Core - Transform server-side validations to client-side without writing any javascript code. (Compatible with Fluent Validation) -
SolidSoils4Arduino
C# .NET - Arduino library supporting simultaneous serial ASCII, Firmata and I2C communication -
Validot
Validot is a performance-first, compact library for advanced model validation. Using a simple declarative fluent interface, it efficiently handles classes, structs, nested members, collections, nullables, plus any relation or combination of them. It also supports translations, custom logic extensions with tests, and DI containers. -
Outcome.NET
Never write a result wrapper again! Outcome.NET is a simple, powerful helper for methods that return a value, but sometimes also need to return validation messages, warnings, or a success bit. -
NaturalSort.Extension
๐ Extension method for StringComparison that adds support for natural sorting (e.g. "abc1", "abc2", "abc10" instead of "abc1", "abc10", "abc2"). -
SystemTextJson.JsonDiffPatch
High-performance, low-allocating JSON object diff and patch extension for System.Text.Json. Support generating patch document in RFC 6902 JSON Patch format. -
trybot
A transient fault handling framework including such resiliency solutions as Retry, Timeout, Fallback, Rate Limit and Circuit Breaker.
WorkOS - The modern identity platform for B2B SaaS
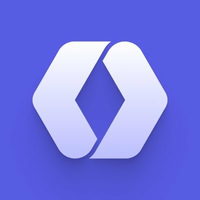
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of Enums.NET or a related project?
README
v4.0 Changes
Removed NonGenericEnums
, NonGenericFlagEnums
, UnsafeEnums
, and UnsafeFlagEnums
classes which were deprecated in v3.0 and also removed all other deprecated methods in an effort to slim the library size down. It is recommended if upgrading from 2.x and below to update to 3.x first and follow the warnings to migrate any code that's using deprecated methods and classes. Also, a dependency on the System.Runtime.CompilerServices.Unsafe
package was added for the .NET 4.5 target in order to remove a build dependency on Fody
.
v3.0 Changes
One of the major changes for v3.0 is the deprecation of the NonGenericEnums
, NonGenericFlagEnums
, UnsafeEnums
, and UnsafeFlagEnums
classes whose methods have been added to the Enums
and FlagEnums
classes to better match System.Enum
and provide better discoverability. To help you migrate your code to using the new methods I have created the C# roslyn analyzer Enums.NET.Analyzer
which provides a code fix to migrate your usages of the non-generic and unsafe methods to the new methods.
Enums.NET
Enums.NET is a high-performance type-safe .NET enum utility library which provides many operations as convenient extension methods. It is compatible with .NET Framework 4.5+ and .NET Standard 1.0+.
What's wrong with System.Enum
- Nearly all of
Enum
's static methods are non-generic leading to the following issues.- Requires the enum type to be explicitly specified as an argument and requires invocation using static method syntax such as
Enum.IsDefined(typeof(ConsoleColor), value)
instead of what should bevalue.IsDefined()
. - Requires casting/unboxing for methods with an enum return value, eg.
ToObject
,Parse
, andGetValues
. - Requires boxing for methods with enum input parameters losing type-safety, eg.
IsDefined
andGetName
.
- Requires the enum type to be explicitly specified as an argument and requires invocation using static method syntax such as
- Support for flag enums is limited to just the
HasFlag
method which isn't type-safe, is inefficient, and is ambiguous as to whether it determines if the value has all or any of the specified flags. It's all by the way. - Most of its methods use reflection on each call without any sort of caching causing poor performance.
- The pattern to associate extra data with an enum member using
Attribute
s is not supported and instead requires users to manually retrieve theAttribute
s via reflection. This pattern is commonly used on enum members with theDescriptionAttribute
,EnumMemberAttribute
, andDisplayAttribute
.
Enums.NET solves all of these issues and more.
Enums.NET Demo
using System;
using System.Linq;
using EnumsNET;
using NUnit.Framework;
using DescriptionAttribute = System.ComponentModel.DescriptionAttribute;
[TestFixture]
class EnumsNETDemo
{
// Enum definitions at bottom
[Test]
public void Enumerate()
{
var count = 0;
// Retrieves all enum members in increasing value order
foreach (var member in Enums.GetMembers<NumericOperator>())
{
NumericOperator value = member.Value;
string name = member.Name;
AttributeCollection attributes = member.Attributes;
++count;
}
Assert.AreEqual(8, count);
count = 0;
// Retrieves distinct values in increasing value order
foreach (var value in Enums.GetValues<NumericOperator>(EnumMemberSelection.Distinct))
{
string name = value.GetName();
AttributeCollection attributes = value.GetAttributes();
++count;
}
Assert.AreEqual(6, count);
}
[Test]
public void FlagEnumOperations()
{
// HasAllFlags
Assert.IsTrue((DaysOfWeek.Monday | DaysOfWeek.Wednesday | DaysOfWeek.Friday).HasAllFlags(DaysOfWeek.Monday | DaysOfWeek.Wednesday));
Assert.IsFalse(DaysOfWeek.Monday.HasAllFlags(DaysOfWeek.Monday | DaysOfWeek.Wednesday));
// HasAnyFlags
Assert.IsTrue(DaysOfWeek.Monday.HasAnyFlags(DaysOfWeek.Monday | DaysOfWeek.Wednesday));
Assert.IsFalse((DaysOfWeek.Monday | DaysOfWeek.Wednesday).HasAnyFlags(DaysOfWeek.Friday));
// CombineFlags ~ bitwise OR
Assert.AreEqual(DaysOfWeek.Monday | DaysOfWeek.Wednesday, DaysOfWeek.Monday.CombineFlags(DaysOfWeek.Wednesday));
Assert.AreEqual(DaysOfWeek.Monday | DaysOfWeek.Wednesday | DaysOfWeek.Friday, FlagEnums.CombineFlags(DaysOfWeek.Monday, DaysOfWeek.Wednesday, DaysOfWeek.Friday));
// CommonFlags ~ bitwise AND
Assert.AreEqual(DaysOfWeek.Monday, DaysOfWeek.Monday.CommonFlags(DaysOfWeek.Monday | DaysOfWeek.Wednesday));
Assert.AreEqual(DaysOfWeek.None, DaysOfWeek.Monday.CommonFlags(DaysOfWeek.Wednesday));
// RemoveFlags
Assert.AreEqual(DaysOfWeek.Wednesday, (DaysOfWeek.Monday | DaysOfWeek.Wednesday).RemoveFlags(DaysOfWeek.Monday));
Assert.AreEqual(DaysOfWeek.None, (DaysOfWeek.Monday | DaysOfWeek.Wednesday).RemoveFlags(DaysOfWeek.Monday | DaysOfWeek.Wednesday));
// GetFlags, splits out the individual flags in increasing significance bit order
var flags = DaysOfWeek.Weekend.GetFlags();
Assert.AreEqual(2, flags.Count);
Assert.AreEqual(DaysOfWeek.Sunday, flags[0]);
Assert.AreEqual(DaysOfWeek.Saturday, flags[1]);
}
[Test]
public new void ToString()
{
// AsString, equivalent to ToString
Assert.AreEqual("Equals", NumericOperator.Equals.AsString());
Assert.AreEqual("-1", ((NumericOperator)(-1)).AsString());
// GetName
Assert.AreEqual("Equals", NumericOperator.Equals.GetName());
Assert.IsNull(((NumericOperator)(-1)).GetName());
// Get description
Assert.AreEqual("Is", NumericOperator.Equals.AsString(EnumFormat.Description));
Assert.IsNull(NumericOperator.LessThan.AsString(EnumFormat.Description));
// Get description if applied, otherwise the name
Assert.AreEqual("LessThan", NumericOperator.LessThan.AsString(EnumFormat.Description, EnumFormat.Name));
}
[Test]
public void Validate()
{
// Standard Enums, checks is defined
Assert.IsTrue(NumericOperator.LessThan.IsValid());
Assert.IsFalse(((NumericOperator)20).IsValid());
// Flag Enums, checks is valid flag combination or is defined
Assert.IsTrue((DaysOfWeek.Sunday | DaysOfWeek.Wednesday).IsValid());
Assert.IsFalse((DaysOfWeek.Sunday | DaysOfWeek.Wednesday | ((DaysOfWeek)(-1))).IsValid());
// Custom validation through IEnumValidatorAttribute<TEnum>
Assert.IsTrue(DayType.Weekday.IsValid());
Assert.IsTrue((DayType.Weekday | DayType.Holiday).IsValid());
Assert.IsFalse((DayType.Weekday | DayType.Weekend).IsValid());
}
[Test]
public void CustomEnumFormat()
{
EnumFormat symbolFormat = Enums.RegisterCustomEnumFormat(member => member.Attributes.Get<SymbolAttribute>()?.Symbol);
Assert.AreEqual(">", NumericOperator.GreaterThan.AsString(symbolFormat));
Assert.AreEqual(NumericOperator.LessThan, Enums.Parse<NumericOperator>("<", ignoreCase: false, symbolFormat));
}
[Test]
public void Attributes()
{
Assert.AreEqual("!=", NumericOperator.NotEquals.GetAttributes().Get<SymbolAttribute>().Symbol);
Assert.IsTrue(Enums.GetMember<NumericOperator>("GreaterThanOrEquals").Attributes.Has<PrimaryEnumMemberAttribute>());
Assert.IsFalse(NumericOperator.LessThan.GetAttributes().Has<DescriptionAttribute>());
}
[Test]
public void Parsing()
{
Assert.AreEqual(NumericOperator.GreaterThan, Enums.Parse<NumericOperator>("GreaterThan"));
Assert.AreEqual(NumericOperator.NotEquals, Enums.Parse<NumericOperator>("1"));
Assert.AreEqual(NumericOperator.Equals, Enums.Parse<NumericOperator>("Is", ignoreCase: false, EnumFormat.Description));
Assert.AreEqual(DaysOfWeek.Monday | DaysOfWeek.Wednesday, Enums.Parse<DaysOfWeek>("Monday, Wednesday"));
Assert.AreEqual(DaysOfWeek.Tuesday | DaysOfWeek.Thursday, FlagEnums.ParseFlags<DaysOfWeek>("Tuesday | Thursday", ignoreCase: false, delimiter: "|"));
}
enum NumericOperator
{
[Symbol("="), Description("Is")]
Equals,
[Symbol("!="), Description("Is not")]
NotEquals,
[Symbol("<")]
LessThan,
[Symbol(">="), PrimaryEnumMember] // PrimaryEnumMember indicates enum member as primary duplicate for extension methods
GreaterThanOrEquals,
NotLessThan = GreaterThanOrEquals,
[Symbol(">")]
GreaterThan,
[Symbol("<="), PrimaryEnumMember]
LessThanOrEquals,
NotGreaterThan = LessThanOrEquals
}
[AttributeUsage(AttributeTargets.Field)]
class SymbolAttribute : Attribute
{
public string Symbol { get; }
public SymbolAttribute(string symbol)
{
Symbol = symbol;
}
}
[Flags]
enum DaysOfWeek
{
None = 0,
Sunday = 1,
Monday = 2,
Tuesday = 4,
Wednesday = 8,
Thursday = 16,
Friday = 32,
Weekdays = Monday | Tuesday | Wednesday | Thursday | Friday,
Saturday = 64,
Weekend = Sunday | Saturday,
All = Sunday | Monday | Tuesday | Wednesday | Thursday | Friday | Saturday
}
[Flags, DayTypeValidator]
enum DayType
{
Weekday = 1,
Weekend = 2,
Holiday = 4
}
[AttributeUsage(AttributeTargets.Enum)]
class DayTypeValidatorAttribute : Attribute, IEnumValidatorAttribute<DayType>
{
public bool IsValid(DayType value) => value.GetFlagCount(DayType.Weekday | DayType.Weekend) == 1 && FlagEnums.IsValidFlagCombination(value);
}
}
[Performance](performance.png)
Results from running the [PerformanceTestConsole](./Src/Enums.NET.PerfTestConsole/Program.cs) BenchmarkDotNet application.
Interface
See fuget for exploring the interface.
Credits
Inspired by Jon Skeet's Unconstrained Melody.