Description
Web Atoms Core is a UI abstraction framework along with powerful MVVM pattern to design modern web and mobile applications.
Web-Atoms Core alternatives and similar packages
Based on the "MVVM" category.
Alternatively, view core alternatives based on common mentions on social networks and blogs.
-
Prism
Prism is a framework for building loosely coupled, maintainable, and testable XAML applications in WPF, Xamarin Forms, and Uno / Win UI Applications.. -
ReactiveUI
An advanced, composable, functional reactive model-view-viewmodel framework for all .NET platforms that is inspired by functional reactive programming. ReactiveUI allows you to abstract mutable state away from your user interfaces, express the idea around a feature in one readable place and improve the testability of your application. -
MVVMCross
The .NET MVVM framework for cross-platform solutions, including Android, iOS, MacCatalyst, macOS, tvOS, WPF, WinUI -
Caliburn.Micro
A small, yet powerful framework, designed for building applications across all XAML platforms. Its strong support for MV* patterns will enable you to build your solution quickly, without the need to sacrifice code quality or testability. -
MVVM Light Toolkit
DISCONTINUED. The main purpose of the toolkit is to accelerate the creation and development of MVVM applications in Xamarin.Android, Xamarin.iOS, Xamarin.Forms, Windows 10 UWP, Windows Presentation Foundation (WPF), Silverlight, Windows Phone. -
Gemini
Gemini is an IDE framework similar in concept to the Visual Studio Shell. It uses AvalonDock and has an MVVM architecture based on Caliburn Micro. -
Stylet
A very lightweight but powerful ViewModel-First MVVM framework for WPF for .NET Framework and .NET Core, inspired by Caliburn.Micro. -
WPF Application Framework (WAF)
Win Application Framework (WAF) is a lightweight Framework that helps you to create well structured XAML Applications. -
FreshMvvm
FreshMvvm is a super light Mvvm Framework designed specifically for Xamarin.Forms. It's designed to be Easy, Simple and Flexible. -
MVVM Dialogs
Library simplifying the concept of opening dialogs from a view model when using MVVM in WPF -
DotNetProjects.WpfToolkit
wpf toolkit fork of the MS WPF Toolkit (https://wpf.codeplex.com/releases/view/40535) -
Smaragd
A platform-independent, lightweight library for developing .NET applications using the MVVM architecture -
Okra App Framework
DISCONTINUED. An app centric MVVM framework for Windows 8.1 built with dependency injection in mind, including a full set of Visual Studio MVVM templates. -
MvvmMicro
A clean and lightweight MVVM framework for WPF, UWP and .NET Standard 2.0 inspired by MVVM Light Toolkit. -
UpdateControls
Update Controls does not require that you implement INotifyPropertyChanged or declare a DependencyProperty. It connects controls directly to CLR properties. This makes it perfect for the Model/View/ViewModel pattern.
WorkOS - The modern identity platform for B2B SaaS
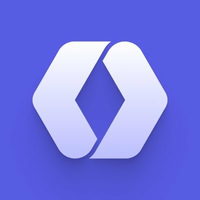
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of Web-Atoms Core or a related project?
README
Web-Atoms Core
Web Atoms Core is a UI abstraction framework along with powerful MVVM pattern to design modern web and mobile applications.
Xamarin.Forms Features
- Use VS Code to develop Xamarin.Forms
- Write TypeScript instead of C#
- Write TSX (JSX) instead of Xaml
- Live hot reload for published app
Web Features
- Abstract Atom Component
- Abstract Device API (Browser Service, Message Broadcast)
- Theme and styles support without CSS
- One time, One way and Two way binding support
- Simple dependency Injection
- In built simple unit testing framework
- UMD module support
- Full featured MVVM Framework with powerful validation
Folder structure
- All views for web must be placed under "web" folder inside "src" folder.
- All views for Xamarin Forms must be placed under "xf" folder inside "src" folder.
Example folder structure
src
+--images
| +--AddButton.svg
|
+--view-Models
| +--TaskListViewModel.ts
| +--TaskEditorViewModel.ts
|
+--web
| +--tasks
| +--TaskListView.tsx
| +--TaskEditorView.tsx
|
+--xf
+--tasks
+--TaskListView.tsx
+--TaskEditorView.tsx
Example View Model
export class UserListViewModel extends AtomViewModel {
public user: any;
public list: IUser[];
public search: string = null;
/// Dependency Injection
@Inject
private listService: ListService;
/// @validate decorator will process this accessor
/// in a way that it will always return null till
/// you call this.isValid. After this.isValid is
/// called, it will display an error if data is invalid
@Validate
public get errorName(): string {
return this.user.name ? "" : "Name cannot be empty";
}
/// You can bind UI element to this field, @watch decorator
/// will process this accessor in a way that UI element bound
/// to this field will automatically update whenever any of
/// fields referenced in this method is updated anywhere else
@Watch
public get name(): string {
return `${this.user.firstName} ${this.user.lastName}`;
}
/// this will be called immediately after the view model
/// has been initialized
/// this will refresh automatically when `this.search` is updated
/// refresh will work for all (this.*.*.*) properties at any level
@Load({ init: true, watch: true })
public async loadItems(ct: CancelToken): Promise<void> {
this.list = await this.listService.loadItems(this.search, ct);
}
/// this will validate all accessors before executing the action
/// and display success message if action was successful
@Action({ validate: true, success: "Added successfully" })
public async addNew(): Promise<any> {
...
}
}
Web Controls
- AtomComboBox (wrapper for SELECT element)
- AtomControl (base class for all other controls)
- AtomItemsControl
- AtomListBox
- AtomPageView (control browser that hosts other control referenced by url)
- AtomWindow
Services
- WindowService - to host AtomWindow and retrieve result
- RestService - RetroFit kind of service for simple ajax
- BrowserService - An abstract navigation service for Web and Xamarin
Development guidelines
- Use TypeScript
module
pattern - Do not use
namespace
- Organize single module in single TypeScript file
- Import only required module and retain naming convention
- Do not define any default export
- No
Atom.get
andAtom.set
- Do not use underscore
_
anywhere, not in field name not in get/set - Do not use
set_name
method name, instead useget name()
andset name(v: T)
syntax for properties.
How to run unit tests?
- Import test class
src\test.ts
- Run
node .\dist\test.js
How to get code coverage?
- Install istanbul,
npm install istanbul --save-dev
- Install remap-istanbul,
npm install remap-istanbul
- Cover Run,
.\node_modules\.bin\istanbul.cmd cover .\dist\test.js
- Report Run,
.\node_modules\.bin\remap-istanbul -i .\coverage\coverage.json -t html -o html-report
- Open generated html-report on browser