Verify alternatives and similar packages
Based on the "Testing" category.
Alternatively, view Verify alternatives based on common mentions on social networks and blogs.
-
Bogus
:card_index: A simple fake data generator for C#, F#, and VB.NET. Based on and ported from the famed faker.js. -
Fluent Assertions
A very extensive set of extension methods that allow you to more naturally specify the expected outcome of a TDD or BDD-style unit tests. Targets .NET Framework 4.7, as well as .NET Core 2.1, .NET Core 3.0, .NET 6, .NET Standard 2.0 and 2.1. Supports the unit test frameworks MSTest2, NUnit3, XUnit2, MSpec, and NSpec3. -
SpecFlow
#1 .NET BDD Framework. SpecFlow automates your testing & works with your existing code. Find Bugs before they happen. Behavior Driven Development helps developers, testers, and business representatives to get a better understanding of their collaboration -
AutoFixture
AutoFixture is an open source library for .NET designed to minimize the 'Arrange' phase of your unit tests in order to maximize maintainability. Its primary goal is to allow developers to focus on what is being tested rather than how to setup the test scenario, by making it easier to create object graphs containing test data. -
Testcontainers
A library to support tests with throwaway instances of Docker containers for all compatible .NET Standard versions. -
NBomber
Modern and flexible load testing framework for Pull and Push scenarios, designed to test any system regardless a protocol (HTTP/WebSockets/AMQP etc) or a semantic model (Pull/Push). -
WireMock.Net
WireMock.Net is a flexible product for stubbing and mocking web HTTP responses using advanced request matching and response templating. Based on the functionality from http://WireMock.org, but extended with more functionality. -
Compare-Net-Objects
What you have been waiting for :+1: Perform a deep compare of any two .NET objects using reflection. Shows the differences between the two objects. -
Machine.Specifications
Machine.Specifications is a Context/Specification framework for .NET that removes language noise and simplifies tests. -
GenFu
GenFu is a library you can use to generate realistic test data. It is composed of several property fillers that can populate commonly named properties through reflection using an internal database of values or randomly created data. You can override any of the fillers, give GenFu hints on how to fill them. -
ArchUnitNET
A C# architecture test library to specify and assert architecture rules in C# for automated testing. -
Expecto
A smooth testing lib for F#. APIs made for humans! Strong testing methodologies for everyone! -
Fine Code Coverage
Visualize unit test code coverage easily for free in Visual Studio Community Edition (and other editions too) -
NFluent
Smooth your .NET TDD experience with NFluent! NFluent is an ergonomic assertion library which aims to fluent your .NET TDD experience (based on simple Check.That() assertion statements). NFluent aims your tests to be fluent to write (with a super-duper-happy 'dot' auto-completion experience), fluent to read (i.e. as close as possible to plain English expression), but also fluent to troubleshoot, in a less-error-prone way comparing to the classical .NET test frameworks. NFluent is also directly inspired by the awesome Java FEST Fluent assertion/reflection library (http://fest.easytesting.org/) -
xBehave.net
DISCONTINUED. ✖ An xUnit.net extension for describing each step in a test with natural language. -
SpecsFor
SpecsFor is a light-weight Behavior-Driven Development framework that focuses on ease of use for *developers* by minimizing testing friction. -
Xunit.Gherkin.Quick
BDD in .NET Core - using Xunit and Gherkin (compatible with both .NET Core and .NET) -
Moq.Contrib.HttpClient
A set of extension methods for mocking HttpClient and IHttpClientFactory with Moq. -
SimpleStubs
*SimpleStubs* is a simple mocking framework that supports Universal Windows Platform (UWP), .NET Core and .NET framework. SimpleStubs is currently developed and maintained by Microsoft BigPark Studios in Vancouver. -
#<Sawyer::Resource:0x00007f89811b9240>
:fire: A small library to help .NET developers leverage Microsoft's dependency injection framework in their Xunit-powered test projects -
SecTester
SecTester is a new tool that integrates our enterprise-grade scan engine directly into your unit tests.
WorkOS - The modern identity platform for B2B SaaS
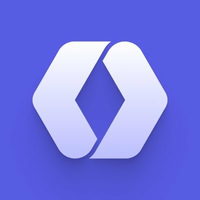
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of Verify or a related project?
Popular Comparisons
README
<!-- GENERATED FILE - DO NOT EDIT This file was generated by MarkdownSnippets. Source File: /readme.source.md To change this file edit the source file and then run MarkdownSnippets. -->
Verify
Verify is a snapshot tool that simplifies the assertion of complex data models and documents.
Verify is called on the test result during the assertion phase. It serializes that result and stores it in a file that matches the test name. On the next test execution, the result is again serialized and compared to the existing file. The test will fail if the two snapshots do not match: either the change is unexpected, or the reference snapshot needs to be updated to the new result.
NuGet packages
- https://nuget.org/packages/Verify.Xunit/
- https://nuget.org/packages/Verify.NUnit/
- https://nuget.org/packages/Verify.Expecto/
- https://nuget.org/packages/Verify.MSTest/
Snapshot management
Accepting or declining a snapshot file is part of the core workflow of Verify. There are several ways to do this and the approach(s) selected is a personal preference.
- In the Windows Tray via DiffEngineTray
- ReSharper test runner support (Source)
- Rider test runner support (Source)
- Via the clipboard.
- Manually making the change in the launched diff tool. Either with a copy paste, or some tools have commands to automate this via a shortcut or a button.
- Manually on the file system. By renaming the
.received.
file to.verified.
. This can be automated via a scripted to bulk accept all (by matching a pattern).received.
files. - Using the dotnet tool Verify.Terminal.
Usage
ImplicitUsings
All examples use ImplicitUsings. Ensure the following is set to have examples compile correctly <ImplicitUsings>enable</ImplicitUsings>
If ImplicitUsings
are not enabled, substitute usages of Verify()
with Verifier.Verify()
.
Class being tested
Given a class to be tested:
<!-- snippet: ClassBeingTested -->
public static class ClassBeingTested
{
public static Person FindPerson() =>
new()
{
Id = new("ebced679-45d3-4653-8791-3d969c4a986c"),
Title = Title.Mr,
GivenNames = "John",
FamilyName = "Smith",
Spouse = "Jill",
Children = new()
{
"Sam",
"Mary"
},
Address = new()
{
Street = "4 Puddle Lane",
Country = "USA"
}
};
}
snippet source | anchor <!-- endSnippet -->
xUnit
Support for xUnit
<!-- snippet: SampleTestXunit -->
[UsesVerify]
public class Sample
{
[Fact]
public Task Test()
{
var person = ClassBeingTested.FindPerson();
return Verify(person);
}
}
snippet source | anchor <!-- endSnippet -->
NUnit
Support for NUnit
<!-- snippet: SampleTestNUnit -->
[TestFixture]
public class Sample
{
[Test]
public Task Test()
{
var person = ClassBeingTested.FindPerson();
return Verify(person);
}
}
snippet source | anchor <!-- endSnippet -->
Expecto
Support for Expecto
<!-- snippet: SampleTestExpecto -->
open Expecto
open VerifyTests
open VerifyExpecto
[<Tests>]
let tests =
testTask "findPerson" {
let person = ClassBeingTested.FindPerson()
do! Verifier.Verify("findPerson", person)
}
snippet source | anchor <!-- endSnippet -->
Caveats
Due to the nature of the Expecto implementation, the following APIs in Verify are not supported.
settings.UseParameters()
settings.UseTextForParameters()
MSTest
Support for MSTest
<!-- snippet: SampleTestMSTest -->
[TestClass]
public class Sample :
VerifyBase
{
[TestMethod]
public Task Test()
{
var person = ClassBeingTested.FindPerson();
return Verify(person);
}
}
snippet source | anchor <!-- endSnippet -->
Initial Verification
No existing .verified.
file.
graph LR
run(Run test and<br/>create Received file)
failTest(Fail Test<br/>and show Diff)
closeDiff(Close Diff)
run-->failTest
shouldAccept{Accept ?}
failTest-->shouldAccept
accept(Move Received<br/>to Verified)
shouldAccept-- Yes -->accept
discard(Discard<br/>Received)
shouldAccept-- No -->discard
accept-->closeDiff
discard-->closeDiff
When the test is initially run will fail. If a Diff Tool is detected it will display the diff.
To verify the result:
- Execute the command from the Clipboard, or
- Accept with DiffEngineTray tool,
- Accept with ReSharper Addin or Rider Addin
- Use the diff tool to accept the changes, or
- Manually copy the text to the new file
Verified result
This will result in the Sample.Test.verified.txt
being created:
<!-- snippet: Verify.Xunit.Tests/Snippets/Sample.Test.verified.txt -->
{
GivenNames: John,
FamilyName: Smith,
Spouse: Jill,
Address: {
Street: 4 Puddle Lane,
Country: USA
},
Children: [
Sam,
Mary
],
Id: Guid_1
}
snippet source | anchor <!-- endSnippet -->
Subsequent Verification
Existing .verified.
file.
graph LR
run(Run test and<br/>create Received file)
closeDiff(Close Diff)
failTest(Fail Test<br/>and show Diff)
run-->isSame
shouldAccept{Accept ?}
failTest-->shouldAccept
accept(Move Received<br/>to Verified)
shouldAccept-- Yes -->accept
discard(Discard<br/>Received)
shouldAccept-- No -->discard
isSame{Compare<br/>Verified +<br/>Received}
passTest(Pass Test and<br/>discard Received)
isSame-- Same --> passTest
isSame-- Different --> failTest
accept-->closeDiff
discard-->closeDiff
If the implementation of ClassBeingTested
changes:
<!-- snippet: ClassBeingTestedChanged -->
public static class ClassBeingTested
{
public static Person FindPerson() =>
new()
{
Id = new("ebced679-45d3-4653-8791-3d969c4a986c"),
Title = Title.Mr,
// Middle name added
GivenNames = "John James",
FamilyName = "Smith",
Spouse = "Jill",
Children = new()
{
"Sam",
"Mary"
},
Address = new()
{
// Address changed
Street = "64 Barnett Street",
Country = "USA"
}
};
}
snippet source | anchor <!-- endSnippet -->
And the test is re run it will fail.
The Diff Tool will display the diff:
The same approach can be used to verify the results and the change to Sample.Test.verified.txt
is committed to source control along with the change to ClassBeingTested
.
VerifyJson
VerifyJson
performs the following actions
- Convert to
JToken
(if necessary). - Apply ignore member by name for keys.
- PrettyPrint the resulting text.
<!-- snippet: VerifyJson -->
[Fact]
public Task VerifyJsonString()
{
var json = "{'key': {'msg': 'No action taken'}}";
return VerifyJson(json);
}
[Fact]
public Task VerifyJsonStream()
{
var json = "{'key': {'msg': 'No action taken'}}";
var stream = new MemoryStream(Encoding.UTF8.GetBytes(json));
return VerifyJson(stream);
}
[Fact]
public Task VerifyJsonJToken()
{
var json = "{'key': {'msg': 'No action taken'}}";
var target = JToken.Parse(json);
return Verify(target);
}
snippet source | anchor <!-- endSnippet -->
Results in:
<!-- snippet: JsonTests.VerifyJsonString.verified.txt -->
{
key: {
msg: No action taken
}
}
snippet source | anchor <!-- endSnippet -->
Source control: Received and Verified files
Received
- All
*.received.*
files should be excluded from source control.
eg. add the following to .gitignore
*.received.*
If using UseSplitModeForUniqueDirectory also include:
*.received/
Verified
All *.verified.*
files should be committed to source control.
All text extensions of *.verified.*
and have eol set to lf
.
eg add the following to .gitattributes
*.verified.txt text eol=lf
*.verified.xml text eol=lf
*.verified.json text eol=lf
Static settings
Most settings are available at the both global level and at the instance level.
When modifying settings at the both global level it should be done using a Module Initializer:
<!-- snippet: StaticSettings.cs -->
[UsesVerify]
public class StaticSettings
{
[Fact]
public Task Test() =>
Verify("String to verify");
}
public static class StaticSettingsUsage
{
[ModuleInitializer]
public static void Initialize() =>
VerifierSettings.AddScrubber(_ => _.Replace("String to verify", "new value"));
}
snippet source | anchor <!-- endSnippet -->
VerifyResult
In some scenarios it can be helpful to get access to the resulting *.verified.*
files after a successful run. For example to do an explicit check for contains or not-contains in the resulting text. To allow this all Verify methods return a VerifyResult
.
<!-- snippet: VerifyResult -->
var result = await Verify(
new
{
Property = "Value To Check"
});
Assert.Contains("Value To Check", result.Text);
snippet source | anchor <!-- endSnippet -->
If using Verifier.Throws
, the resulting Exception
will also be accessible
<!-- snippet: ExceptionResult -->
var result = await Verifier.Throws(MethodThatThrows);
Assert.NotNull(result.Exception);
snippet source | anchor <!-- endSnippet -->
CurrentFile
Utility for finding paths based on the current file.
<!-- snippet: CurrentFile.cs -->
using IOPath = System.IO.Path;
namespace VerifyTests;
public static class CurrentFile
{
public static string Path([CallerFilePath] string file = "") =>
file;
public static string Directory([CallerFilePath] string file = "") =>
IOPath.GetDirectoryName(file)!;
public static string Relative(string relative, [CallerFilePath] string file = "")
{
var directory = IOPath.GetDirectoryName(file)!;
return IOPath.Combine(directory, relative);
}
}
snippet source | anchor <!-- endSnippet -->
Versioning
Verify follows Semantic Versioning. The same applies for extensions to Verify. Small changes in the resulting snapshot files may be deployed in a minor version. As such nuget updates to Verify.*
should be done as follows:
- Updates all
Verify.*
packages in isolation - Re-run all tests.
- If there are changes, ensure they look correct given the release notes. If the changes do not look correct, raise an issue.
- Accept those changes.
Snapshot changes do not trigger a major version change to avoid causing Diamond dependency issues for downstream extensions.
Media
- Unit testing assertions are now easier than ever with Verify Snapshot tool - Rana Krishnrajsinh (20 October 2022)
- Testing C# code reliably by freezing it in time - Nick Chapsas (1 August 2022)
- Snapshot Testing in .NET with Verify - Dan Clarke (21 July 2022)
- Testing an incremental generator with snapshot testing (14 Dec 2021)
- Snapshot Testing with Verify - Dan Clarke (10 Dec 2021)
- OSS Power-Ups: Verify (14 Jul 2021)
- Unhandled Exception podcast: Snapshot Testing (26 Nov 2021)
- 5 helpful Nuget package for Unit Testing in .NET (16 Oct 2021)
- 5 open source .NET projects that deserve more attention (9 Sep 2021)
- Verify: Snapshot Testing for C# (23rd Nov. 2020)
- Verify Xunit Intro (26 Apr 2020)
Extensions
- Verify.AngleSharp: Html verification utilities via AngleSharp.
- Verify.AspNetCore: Verification of AspNetCore bits.
- Verify.Aspose: Verification of documents (pdf, docx, xslx, and pptx) via Aspose.
- Verify.Blazor: Verification of Blazor Component via bunit or via raw Blazor rendering.
- Verify.Cosmos: Verification of Azure CosmosDB.
- Verify.DiffPlex: Comparison of text via DiffPlex.
- Verify.DocNet: Verification of pdfs via DocNet.
- Verify.EntityFramework: Verification of EntityFramework bits.
- Verify.FakeItEasy: Verification of FakeItEasy bits.
- Verify.HeadlessBrowsers: Verification of Web UIs using Playwright, Puppeteer Sharp, or Selenium.
- Verify.Http: Verification of Http bits.
- Verify.ICSharpCode.Decompiler: Comparison of assemblies and types via ICSharpCode.Decompiler.
- Verify.ImageHash: Comparison of images via ImageHash.
- Verify.ImageSharp.Compare: Verification and comparison of images via Codeuctivity.ImageSharp.Compare.
- Verify.ImageMagick: Verification and comparison of images via Magick.NET.
- Verify.ImageSharp: Verification of images via ImageSharp.
- Verify.MassTransit: Support for MassTransit test helpers.
- Verify.MicrosoftLogging: Verify MicrosoftLogging.
- Verify.MongoDB: Verification of MongoDB bits.
- Verify.Moq: Verification of Moq bits.
- Verify.NodaTime: Support for NodaTime.
- Verify.NewtonsoftJson: Support for converting Newtonsoft.Json types (JObject and JArray).
- Verify.NServiceBus: Verify NServiceBus Test Contexts.
- Verify.NSubstitute: Support for NSubstitute types
- Verify.PdfPig: Verification of pdfs via PdfPig.
- Verify.Phash: Comparison of images via Phash.
- Verify.Quibble: Comparison of objects via Quibble.
- Verify.QuestPDF: Verification of pdf documents via QuestPDF.
- Verify.RavenDb: Verification of RavenDb bits.
- Verify.Serilog: Verification of Serilog bits.
- Verify.SqlServer: Verification of SqlServer bits.
- Verify.SourceGenerators: Verification of C# Source Generators.
- Verify.SystemJson: Support for converting System.Text.Json types.
- Verify.Terminal: A dotnet tool for managing Verify snapshots.
- Verify.WinForms: Verification of WinForms UIs.
- Verify.Xamarin: Verification of Xamarin UIs.
- Verify.Xaml: Verification of Xaml UIs.
- Spectre.Verify.Extensions: Add an attribute driven file naming convention to Verify.
- Verify.Syncfusion: Verification of documents (pdf, docx, xslx, and pptx) via Syncfusion File Formats.
More Documentation
- Clipboard <!-- include: doc-index. path: /docs/mdsource/doc-index.include.md -->
- Compared to assertions
- Verify options
- VerifyDirectory
- VerifyFile
- VerifyXml
- Serializer Settings
- File naming
- Parameterised tests
- Named Tuples
- Scrubbers
- Diff Engine
- Diff Tools
- Diff Tool Order
- Custom Diff Tool
- Using anonymous types
- Verifying binary data
- Build server
- Comparers
- Converters
- Explicit Targets
- FSharp Usage
- Compared to ApprovalTests <!-- endInclude -->
Icon
Helmet designed by Leonidas Ikonomou from The Noun Project.