Description
Xunit.Gherkin.Quick is a lightweight, cross platform BDD test framework (targets .NET Standard, can be used from both .NET and .NET Core test projects). It parses Gherkin language and executes Xunit tests corresponding to scenarios.
Xunit.Gherkin.Quick alternatives and similar packages
Based on the "Testing" category.
Alternatively, view Xunit.Gherkin.Quick alternatives based on common mentions on social networks and blogs.
-
Bogus
:card_index: A simple fake data generator for C#, F#, and VB.NET. Based on and ported from the famed faker.js. -
Fluent Assertions
A very extensive set of extension methods that allow you to more naturally specify the expected outcome of a TDD or BDD-style unit tests. Targets .NET Framework 4.7, as well as .NET Core 2.1, .NET Core 3.0, .NET 6, .NET Standard 2.0 and 2.1. Supports the unit test frameworks MSTest2, NUnit3, XUnit2, MSpec, and NSpec3. -
SpecFlow
#1 .NET BDD Framework. SpecFlow automates your testing & works with your existing code. Find Bugs before they happen. Behavior Driven Development helps developers, testers, and business representatives to get a better understanding of their collaboration -
AutoFixture
AutoFixture is an open source library for .NET designed to minimize the 'Arrange' phase of your unit tests in order to maximize maintainability. Its primary goal is to allow developers to focus on what is being tested rather than how to setup the test scenario, by making it easier to create object graphs containing test data. -
Testcontainers
A library to support tests with throwaway instances of Docker containers for all compatible .NET Standard versions. -
Verify
Verify is a snapshot tool that simplifies the assertion of complex data models and documents. -
NBomber
Modern and flexible load testing framework for Pull and Push scenarios, designed to test any system regardless a protocol (HTTP/WebSockets/AMQP etc) or a semantic model (Pull/Push). -
WireMock.Net
WireMock.Net is a flexible product for stubbing and mocking web HTTP responses using advanced request matching and response templating. Based on the functionality from http://WireMock.org, but extended with more functionality. -
Compare-Net-Objects
What you have been waiting for :+1: Perform a deep compare of any two .NET objects using reflection. Shows the differences between the two objects. -
Machine.Specifications
Machine.Specifications is a Context/Specification framework for .NET that removes language noise and simplifies tests. -
GenFu
GenFu is a library you can use to generate realistic test data. It is composed of several property fillers that can populate commonly named properties through reflection using an internal database of values or randomly created data. You can override any of the fillers, give GenFu hints on how to fill them. -
ArchUnitNET
A C# architecture test library to specify and assert architecture rules in C# for automated testing. -
Expecto
A smooth testing lib for F#. APIs made for humans! Strong testing methodologies for everyone! -
Fine Code Coverage
Visualize unit test code coverage easily for free in Visual Studio Community Edition (and other editions too) -
NFluent
Smooth your .NET TDD experience with NFluent! NFluent is an ergonomic assertion library which aims to fluent your .NET TDD experience (based on simple Check.That() assertion statements). NFluent aims your tests to be fluent to write (with a super-duper-happy 'dot' auto-completion experience), fluent to read (i.e. as close as possible to plain English expression), but also fluent to troubleshoot, in a less-error-prone way comparing to the classical .NET test frameworks. NFluent is also directly inspired by the awesome Java FEST Fluent assertion/reflection library (http://fest.easytesting.org/) -
xBehave.net
DISCONTINUED. ✖ An xUnit.net extension for describing each step in a test with natural language. -
SpecsFor
SpecsFor is a light-weight Behavior-Driven Development framework that focuses on ease of use for *developers* by minimizing testing friction. -
SimpleStubs
*SimpleStubs* is a simple mocking framework that supports Universal Windows Platform (UWP), .NET Core and .NET framework. SimpleStubs is currently developed and maintained by Microsoft BigPark Studios in Vancouver. -
Moq.Contrib.HttpClient
A set of extension methods for mocking HttpClient and IHttpClientFactory with Moq. -
#<Sawyer::Resource:0x00007f89811b9240>
:fire: A small library to help .NET developers leverage Microsoft's dependency injection framework in their Xunit-powered test projects -
SecTester
SecTester is a new tool that integrates our enterprise-grade scan engine directly into your unit tests.
WorkOS - The modern identity platform for B2B SaaS
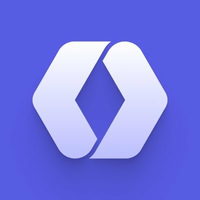
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of Xunit.Gherkin.Quick or a related project?
README
Xunit.Gherkin.Quick
Xunit.Gherkin.Quick is a lightweight, cross platform BDD test framework (targets .NET Standard, can be used from both .NET and .NET Core test projects). It parses Gherkin language and executes Xunit tests corresponding to scenarios.
Key differences of Xunit.Gherkin.Quick from other BDD frameworks are:
- You write your expectations in a human-readable Gherkin language, not in code. This approach allows separation of concerns and responsibilities between requirement and code writing.
- Xunit.Gherkin.Quick is a lightweight framework. No auto-generated code that you fear to change; no Visual Studio dependency where the auto-generation works. With Xunit.Gherkin.Quick, you don't even need an IDE (althought it works conveniently in Visual Studio) - one could write feature text files in a notepad, and code in any dev plugin; then run tests via .NET Core CLI.
- Supports full spectrum of Gherkin language idioms such as Given/When/Then/And/But/* keywords, Scenario Outlines, Scenario Backgrounds, and native Gherkin argument types (DocString, DataTable).
- All supported features are fully documented. Scroll down to the Documentation and Reference section after going through the Getting Started topic on this page.
Enjoy coding!
Table of Contents
Project Sponsors
Why sponsor? Some organizations might want to have premium support while using the framework; others might want to prioritize implementing certain new capabilities (if they fit within the framework's vision and roadmap). If you wish to be a sponsor, please find the sponsoring link on this page. Feel free to contact me for more details.
This section will showcase the sponsors' logos, if they wish to do so.
Getting Started
Prefer video format? Watch how to get started with BDD and Xunit.Gherkin.Quick on Youtube.
We'll quickly setup our project, write our first BDD test, and then run it.
Xunit Test Project
Create a new or open existing Xunit test project. Xunit.Gherkin.Quick
needs to be used with Xunit.
Install Nuget Package
Package name to search for through GUI: Xunit.Gherkin.Quick
Package Manager:
Install-Package Xunit.Gherkin.Quick
.NET Core:
dotnet add package Xunit.Gherkin.Quick
These steps should take care of the installation, but if you need more info about setup or the nuget package, click here: https://www.nuget.org/packages/Xunit.Gherkin.Quick/
Create Gherkin Feature File
Create a new text file. Name it as AddTwoNumbers.feature
.
Important: change feature file properties to ensure it gets copied into output directory. Set the value of Copy to Output Directory
to Copy Always or Copy if Newer. See Copying Feature Files for more options.
NOTE: In practice, you can name your files in any way you want, and .feature extension is not necessary either.
Copy the below code and paste it into your feature file:
Feature: AddTwoNumbers
In order to learn Math
As a regular human
I want to add two numbers using Calculator
Scenario: Add two numbers
Given I chose 12 as first number
And I chose 15 as second number
When I press add
Then the result should be 27 on the screen
This is a BDD style feature written in Gherkin language.
Now it's time to implement the code to run scenarios of this feature.
Note: at this point, the new feature file's scenarios will not be discovered by the test runner either via Visual Studio or via the command line execution. By default, you need to have a corresponding feature class in your project which refers to this new feature file. If you want to instead see every new feature file's scenarios right after they are added without the necessity to have the corresponding feature class, please see Handling Not-Implemented Feature Files.
Implement Feature Scenario
Implementing a scenario simply means writing methods in the Feature
-derived class. Goal is to ensure that each scenario step above will match a method by using regex syntax. If we miss a step and it does not match a method, we will receive an error when we try to run the scenario test.
Here is how we can implement the scenario of the above feature:
[FeatureFile("./Addition/AddTwoNumbers.feature")]
public sealed class AddTwoNumbers : Feature
{
private readonly Calculator _calculator = new Calculator();
[Given(@"I chose (\d+) as first number")]
public void I_chose_first_number(int firstNumber)
{
_calculator.SetFirstNumber(firstNumber);
}
[And(@"I chose (\d+) as second number")]
public void I_chose_second_number(int secondNumber)
{
_calculator.SetSecondNumber(secondNumber);
}
[When(@"I press add")]
public void I_press_add()
{
_calculator.AddNumbers();
}
[Then(@"the result should be (\d+) on the screen")]
public void The_result_should_be_z_on_the_screen(int expectedResult)
{
var actualResult = _calculator.Result;
Assert.Equal(expectedResult, actualResult);
}
}
Notice a couple of things:
FeatureFile
attribute for the class refers to the feature file location (relative to the project root, not relative to the class file). You don't need to apply this attribute if you keep your feature files in the root directory of your project, because that's where it will be located by default. Buf if you keep it under a sub-folder (which I do), then make sure to specify the file location (either relative or absolute) using this attribute.Given
,When
andThen
attributes specify scenario step text which they need to match. If you want to extract value from the text, you need to use parentheses. Behind the scenes this is done using .NET Regex syntax. Regex group values are passed as argument values. You can also useAnd
andBut
attributes, which work similarly.Scenario step method can be
async
, or can be a regular method, just as shown in the example above.
Run Scenario
Build BDD tests project.
If you use command line to run unit tests, simply run them as always (e.g., run dotnet test
command inside a solution or test project folder). You should see the scenario full name in the results as a newly added unit test name.
If you use Visual Studio to run unit tests, open Test Explorer to see the new test item (important: use built-in Test Explorer, not ReSharper or anything else). Right click and run it as you would usually run unit tests through test explorer.
Unit test name in this case will be "AddTwoNumbers :: Add two numbers", which is a combination of feature name "AddTwoNumbers" and scenario name "Add two numbers".
[Screenshot of scenario test run](scenario-test-run-screenshot.png)
Add More Scenarios
If the feature has multiple scenarios, add them to the same feature file. They will show up as additional tests in the test explorer. And they will need additional methods in the same feature class for execution.
Got Stuck?
Look into our issues if your problem was already resolved. Also try searching StackOverflow.
Feel free to post your question/problem either into our issues repository, or into StackOverflow.
Check out a fully-working sample project with BDD test implementations for every supported feature: ProjectConsumer.
Special Thanks
I want to send special Thank You to all the contributors, which you can see here: https://github.com/ttutisani/Xunit.Gherkin.Quick/graphs/contributors
Documentation and Reference
- Handling Not-Implemented Feature Files
- Step Attributes (Given, When, Then, And, But) usage instructions
- DataTable Argument usage instructions
- DocString (multi-line text) Argument usage instructions
- Gherking Tags usage instructions
- Scenario Outline usage instructions
- Scenario Background usage instructions
- Logging Output from Scenario Steps to Standard Test Output
- Ignoring Scenario, Scenario Outline, Examples, or Feature
- Star Notation Support - How to Skip Keywords in Scenario Steps
- Shared Step Method With Multiple Step Attributes
- Reusing step implementation across features
- Before and After Scenario Execution Hooks
- Syntax highlighting of Gherkin/Cucumber
- Integrating Test Results With PicklesDoc
- Copying feature files
- Feature file character encoding
- Domain Model (for contributors)