Description
The DatabaseObjects library is .NET object relational mapping tool that contains a large set of powerful classes that interface to the underlying database system. Classes and properties in the business layer / model are marked with attributes (or functions overridden) in order to indicate the table and field mappings to the database. The library is simple, powerful and easy to learn. There are no external files to setup - everything is setup using standard object-oriented techniques. It is light-weight, flexible and provides facilities for ensuring maximum performance. It is also under active development and requests for changes are always welcome.
DatabaseObjects alternatives and similar packages
Based on the "ORM" category.
Alternatively, view DatabaseObjects alternatives based on common mentions on social networks and blogs.
-
TypeORM
ORM for TypeScript and JavaScript. Supports MySQL, PostgreSQL, MariaDB, SQLite, MS SQL Server, Oracle, SAP Hana, WebSQL databases. Works in NodeJS, Browser, Ionic, Cordova and Electron platforms. -
Dapper
DISCONTINUED. Dapper - a simple object mapper for .Net [Moved to: https://github.com/DapperLib/Dapper] -
Entity Framework
EF Core is a modern object-database mapper for .NET. It supports LINQ queries, change tracking, updates, and schema migrations. -
SqlSugar
.Net aot ORM Fastest ORM Simple Easy Sqlite orm Oracle ORM Mysql Orm postgresql ORm SqlServer oRm 达梦 ORM 人大金仓 ORM 神通ORM C# ORM , C# ORM .NET ORM NET5 ORM .NET6 ORM ClickHouse orm QuestDb ,TDengine ORM,OceanBase orm,GaussDB orm ,Tidb orm Object/Relational Mapping -
FreeSql
🦄 .NET aot orm, C# orm, VB.NET orm, Mysql orm, Postgresql orm, SqlServer orm, Oracle orm, Sqlite orm, Firebird orm, 达梦 orm, 人大金仓 orm, 神通 orm, 翰高 orm, 南大通用 orm, 虚谷 orm, 国产 orm, Clickhouse orm, QuestDB orm, MsAccess orm. -
EFCore.BulkExtensions
Entity Framework EF Core efcore Bulk Batch Extensions with BulkCopy in .Net for Insert Update Delete Read (CRUD), Truncate and SaveChanges operations on SQL Server, PostgreSQL, MySQL, SQLite -
Dapper Extensions
Dapper Extensions is a small library that complements Dapper by adding basic CRUD operations (Get, Insert, Update, Delete) for your POCOs. For more advanced querying scenarios, Dapper Extensions provides a predicate system. The goal of this library is to keep your POCOs pure by not requiring any attributes or base class inheritance. -
Entity Framework 6
This is the codebase for Entity Framework 6 (previously maintained at https://entityframework.codeplex.com). Entity Framework Core is maintained at https://github.com/dotnet/efcore. -
SmartSql
SmartSql = MyBatis in C# + .NET Core+ Cache(Memory | Redis) + R/W Splitting + PropertyChangedTrack +Dynamic Repository + InvokeSync + Diagnostics -
NPoco
Simple microORM that maps the results of a query onto a POCO object. Project based on Schotime's branch of PetaPoco -
SQLProvider
A general F# SQL database erasing type provider, supporting LINQ queries, schema exploration, individuals, CRUD operations and much more besides. -
MongoDB.Entities
A data access library for MongoDB with an elegant api, LINQ support and built-in entity relationship management -
MongoDB Repository pattern implementation
DISCONTINUED. Repository abstraction layer on top of Official MongoDB C# driver -
DbExtensions
Data-access framework with a strong focus on query composition, granularity and code aesthetics. -
NReco.Data
Fast DB-independent DAL for .NET Core: abstract queries, SQL commands builder, schema-less data access, POCO mapping (micro-ORM). -
EntityFrameworkCore.SqlServer.SimpleBulks
Very simple .net library that supports bulk insert (retain client populated Ids or return db generated Ids), bulk update, bulk delete and bulk merge operations. Lambda Expression is supported. -
Linq.Expression.Optimizer
System.Linq.Expression expressions optimizer. http://thorium.github.io/Linq.Expression.Optimizer -
EntityFramework.DatabaseMigrator
EntityFramework.DatabaseMigrator is a WinForms utility to help manage Entity Framework 6.0+ migrations.
InfluxDB - Power Real-Time Data Analytics at Scale
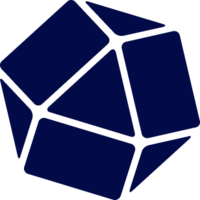
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of DatabaseObjects or a related project?
README
DatabaseObjects
Database Support
- SQL Server
- MySQL
- SQLite
- HyperSQL
- Microsoft Access
- Pervasive
Environment Support
- Windows
- iOS via MonoTouch
- Android via MonoDroid
- OSX / Linux via Mono
Overview
The DatabaseObjects library is .NET object relational mapping tool that contains a large set of powerful classes that interface to the underlying database system. Classes and properties in the business layer / model are marked with attributes (or functions overridden) in order to indicate the table and field mappings to the database. The library is simple, powerful and easy to learn. There are no external files to setup - everything is setup using standard object-oriented techniques. It is light-weight, flexible and provides facilities for ensuring maximum performance. It is also under active development and requests for changes are always welcome.
License
Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License.
SQL Support
The library also supports other database commands for creating / altering tables, indexes, views and specifying table joins, arithmetic expressions, aggregates, grouping, ordering, unions and support for all common data types and more. All commands are database agnostic.
Videos
A series of tutorial videos are available [here].(http://www.youtube.com/user/80twix/)
Documentation
All documentation and other information is available on the website www.hisystems.com.au/databaseobjects
Example
Below is a really simple example of what the library can do. This is really just a taste, see the demo project or the unit test project for more advanced examples.
namespace Northwind.Model
{
public class Northwind
{
public Suppliers Suppliers { get; private set; }
public Northwind()
{
var database = new DatabaseObjects.MicrosoftSQLServerDatabase("localhost", "Northwind");
this.Suppliers = new Suppliers(database);
}
}
[DatabaseObjects.Table("Suppliers")]
[DatabaseObjects.DistinctField("SupplierID", eAutomaticAssignment: DatabaseObjects.SQL.FieldValueAutoAssignmentType.AutoIncrement)]
[DatabaseObjects.OrderByField("CompanyName")]
public class Suppliers : DatabaseObjects.Generic.DatabaseObjectsEnumerable<Supplier>
{
internal Suppliers(DatabaseObjects.Database database)
: base(database)
{
}
}
public class Supplier : DatabaseObjects.DatabaseObject
{
[DatabaseObjects.FieldMapping("CompanyName")]
public string Name { get; set; }
[DatabaseObjects.FieldMapping("ContactName")]
public string ContactName { get; set; }
internal Supplier(Suppliers suppliers)
: base(suppliers)
{
}
}
}
namespace Northwind.Executable
{
using Model;
public class Program
{
public static void Main(string[] args)
{
var model = new Northwind();
foreach (Supplier supplier in model.Suppliers)
System.Console.WriteLine(supplier.Name);
}
}
}
Demo Project
The demonstration project is available in a separate repository on Github here. It includes a number of examples on how to utilise the library. To run the demo project in conjunction with the library it must be located in same directory as the library.
For example:
/DatabaseObjects /DatabaseObjects.Demo
Unit Test Project
The unit test project is available in a separate repository on Github here. It is also a good resource for examples on how to utilise the library. To run the unit tests project in conjunction with the library it must be located in the same directory as the library.
For example:
/DatabaseObjects /DatabaseObjects.UnitTests
*Note that all licence references and agreements mentioned in the DatabaseObjects README section above
are relevant to that project's source code only.