Description
This is a library for importing Excel spreadsheets into SQL Server tables. In a nutshell, use the `ExcelLoader` class and call one of the `Save` overloads accepting a filename or `Stream`. Customize with ability to add custom columns, removing whitespace and non-printing characters.
Excel2SqlServer alternatives and similar packages
Based on the "ORM" category.
Alternatively, view Excel2SqlServer alternatives based on common mentions on social networks and blogs.
-
TypeORM
ORM for TypeScript and JavaScript. Supports MySQL, PostgreSQL, MariaDB, SQLite, MS SQL Server, Oracle, SAP Hana, WebSQL databases. Works in NodeJS, Browser, Ionic, Cordova and Electron platforms. -
Dapper
DISCONTINUED. Dapper - a simple object mapper for .Net [Moved to: https://github.com/DapperLib/Dapper] -
Entity Framework
EF Core is a modern object-database mapper for .NET. It supports LINQ queries, change tracking, updates, and schema migrations. -
SqlSugar
.Net aot ORM Fastest ORM Simple Easy Sqlite orm Oracle ORM Mysql Orm postgresql ORm SqlServer oRm 达梦 ORM 人大金仓 ORM 神通ORM C# ORM , C# ORM .NET ORM NET5 ORM .NET6 ORM ClickHouse orm QuestDb ,TDengine ORM,OceanBase orm,GaussDB orm ,Tidb orm Object/Relational Mapping -
FreeSql
🦄 .NET aot orm, C# orm, VB.NET orm, Mysql orm, Postgresql orm, SqlServer orm, Oracle orm, Sqlite orm, Firebird orm, 达梦 orm, 人大金仓 orm, 神通 orm, 翰高 orm, 南大通用 orm, 虚谷 orm, 国产 orm, Clickhouse orm, QuestDB orm, MsAccess orm. -
EFCore.BulkExtensions
Entity Framework EF Core efcore Bulk Batch Extensions with BulkCopy in .Net for Insert Update Delete Read (CRUD), Truncate and SaveChanges operations on SQL Server, PostgreSQL, MySQL, SQLite -
Dapper Extensions
Dapper Extensions is a small library that complements Dapper by adding basic CRUD operations (Get, Insert, Update, Delete) for your POCOs. For more advanced querying scenarios, Dapper Extensions provides a predicate system. The goal of this library is to keep your POCOs pure by not requiring any attributes or base class inheritance. -
Entity Framework 6
This is the codebase for Entity Framework 6 (previously maintained at https://entityframework.codeplex.com). Entity Framework Core is maintained at https://github.com/dotnet/efcore. -
NPoco
Simple microORM that maps the results of a query onto a POCO object. Project based on Schotime's branch of PetaPoco -
SmartSql
SmartSql = MyBatis in C# + .NET Core+ Cache(Memory | Redis) + R/W Splitting + PropertyChangedTrack +Dynamic Repository + InvokeSync + Diagnostics -
SQLProvider
A general F# SQL database erasing type provider, supporting LINQ queries, schema exploration, individuals, CRUD operations and much more besides. -
MongoDB.Entities
A data access library for MongoDB with an elegant api, LINQ support and built-in entity relationship management -
MongoDB Repository pattern implementation
DISCONTINUED. Repository abstraction layer on top of Official MongoDB C# driver -
DbExtensions
Data-access framework with a strong focus on query composition, granularity and code aesthetics. -
NReco.Data
Fast DB-independent DAL for .NET Core: abstract queries, SQL commands builder, schema-less data access, POCO mapping (micro-ORM). -
EntityFrameworkCore.SqlServer.SimpleBulks
Very simple .net library that supports bulk insert (retain client populated Ids or return db generated Ids), bulk update, bulk delete and bulk merge operations. Lambda Expression is supported. -
Linq.Expression.Optimizer
System.Linq.Expression expressions optimizer. http://thorium.github.io/Linq.Expression.Optimizer
WorkOS - The modern identity platform for B2B SaaS
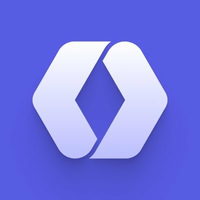
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of Excel2SqlServer or a related project?
README
This is a library for importing Excel spreadsheets into SQL Server tables using Excel Data Reader.
Nuget package: Excel2SqlServer
In a nutshell, use the ExcelLoader class and call one of the SaveAsync
overloads. You can use a local filename or a stream as input. Here's a simple example that loads a single table from a local file:
using (var cn = GetConnection())
{
var loader = new ExcelLoader();
await loader.SaveAsync("MyFile.xlsx", cn, "dbo", "MyTable");
}
This will save an Excel file called MyFile.xlsx
to a database table dbo.MyTable
. The table is created if it doesn't exist. Note also there is an int identity(1,1)
column created called Id
if it doesn't already exist in the spreadsheet.
If a spreadsheet has multiple sheets and you want to import all the sheets into multiple tables, omit the schema and table name from the SaveAsync
call. ExcelLoader
will use the sheet names in the spreadsheet to build the table names. If you need to customize the table names, you can pass a Dictionary<string, ObjectName>
where the key represents the sheet name, and the ObjectName
is the schema + object of the resulting table.
By default, data is always appended to existing data. You can pass an optional Options object to customize the load behavior. For example:
using (var stream = await blob.OpenReadAsync())
{
using (var cn = GetConnection())
{
var loader = new ExcelLoader();
int rows = await loader.SaveAsync(stream, cn, "dbo", "MyTable", new Options()
{
TruncateFirst = true,
AutoTrimStrings = true,
RemoveNonPrintingChars = true,
CustomColumns = new string[]
{
"[IsProcessed] bit NOT NULL DEFAULT (0)",
"[DateUploaded] datetime NOT NULL DEFAULT getdate()"
}
});
}
}
This will append some extra columns to the table when it's created IsProcessed
and DateUploaded
.
An encoding error you might see
Note, if you see an error like this...
...try adding this line before you use ExcelLoader
:
Encoding.RegisterProvider(CodePagesEncodingProvider.Instance);
Reference
- Task CreateTableAsync (string fileName, SqlConnection connection, string schemaName, string tableName, [ IEnumerable customColumns ])
- Task CreateTableAsync (Stream stream, SqlConnection connection, string schemaName, string tableName, [ IEnumerable customColumns ])
- Task<int> SaveAsync (string fileName, SqlConnection connection, [ Dictionary tableNames ], [ Options options ])
- Task<int> SaveAsync (string fileName, SqlConnection connection, string schemaName, string tableName, [ Options options ])
- Task<int> SaveAsync (Stream stream, SqlConnection connection, [ Dictionary tableNames ], [ Options options ])
- Task<int> SaveAsync (Stream stream, SqlConnection connection, string schemaName, string tableName, [ Options options ])
- Task<DataSet> ReadAsync (string fileName)
- Task<DataSet> ReadAsync (Stream stream)